Seat Booking System: Eusoff DP1516
This is a seat booking system for Eusoff Hall (NUS) dance production 15/16. They would like to sell tickets for their performance in UCC (University Culture Center NUS) and there was a need for the booking system. I participated in building this system and learnt a lot in doing this.
Overall Booking Process
Step 1
Go to the booking page:
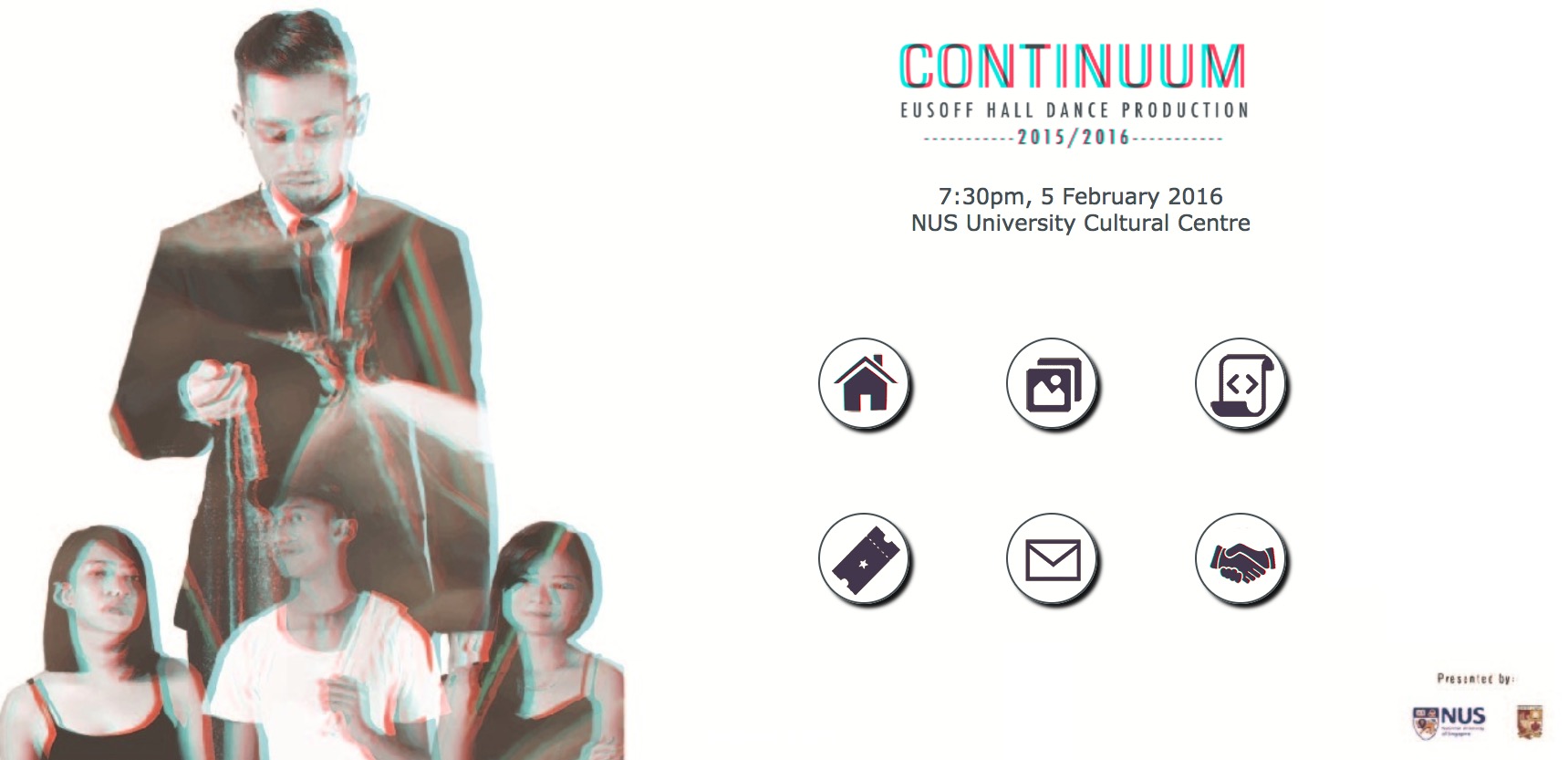
Step 2
Select a seats:
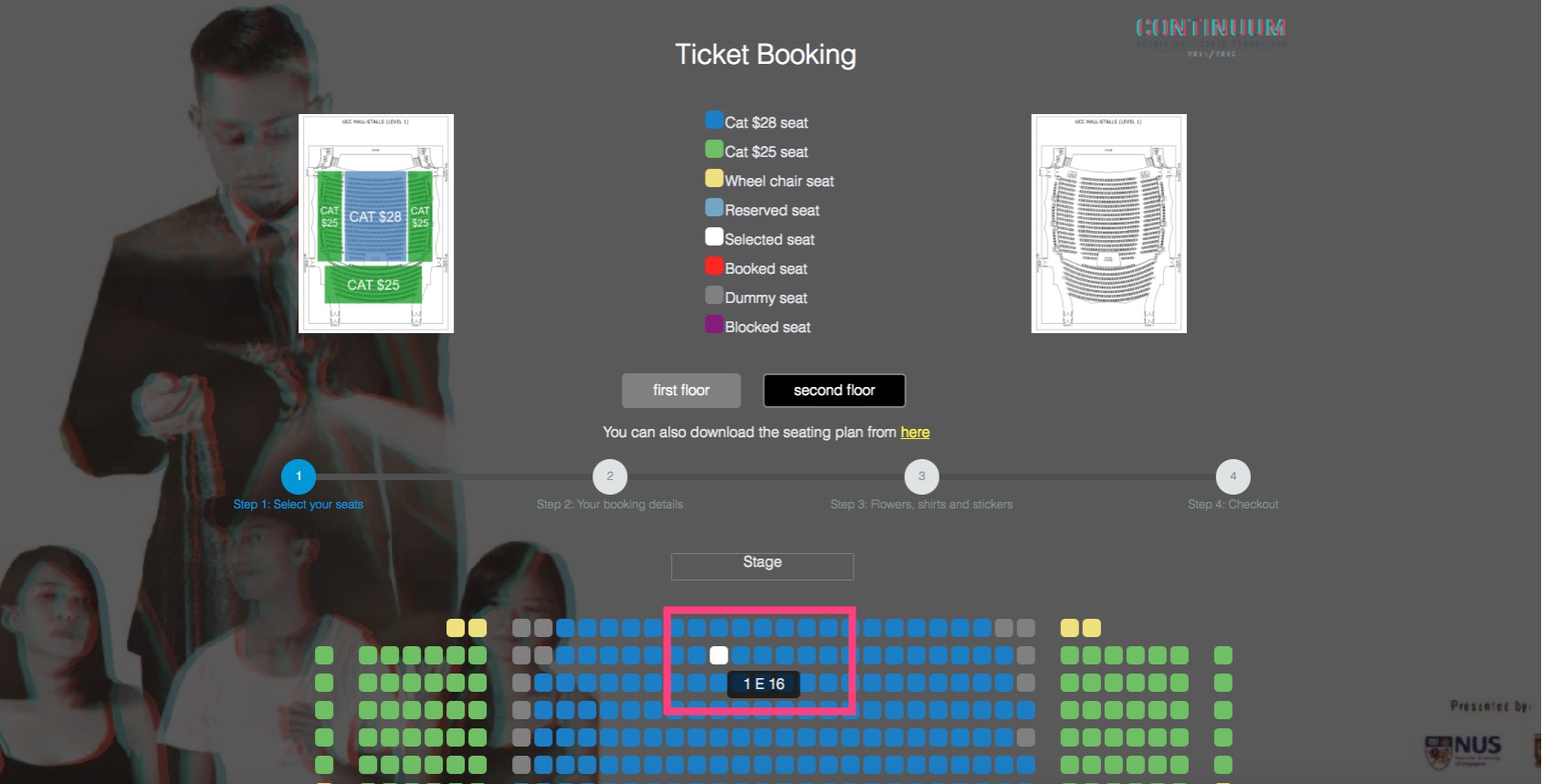
- The seat selected will be blocked by sending AJAX query to the sever
- A summary of booking details will be shown below

Also, you cannot leave a seat between :P
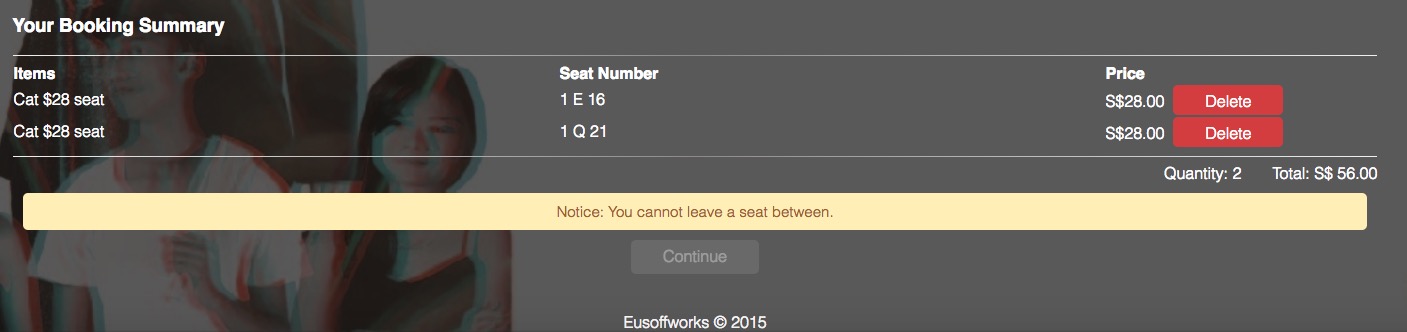
Code for checking seat between.
Step 3
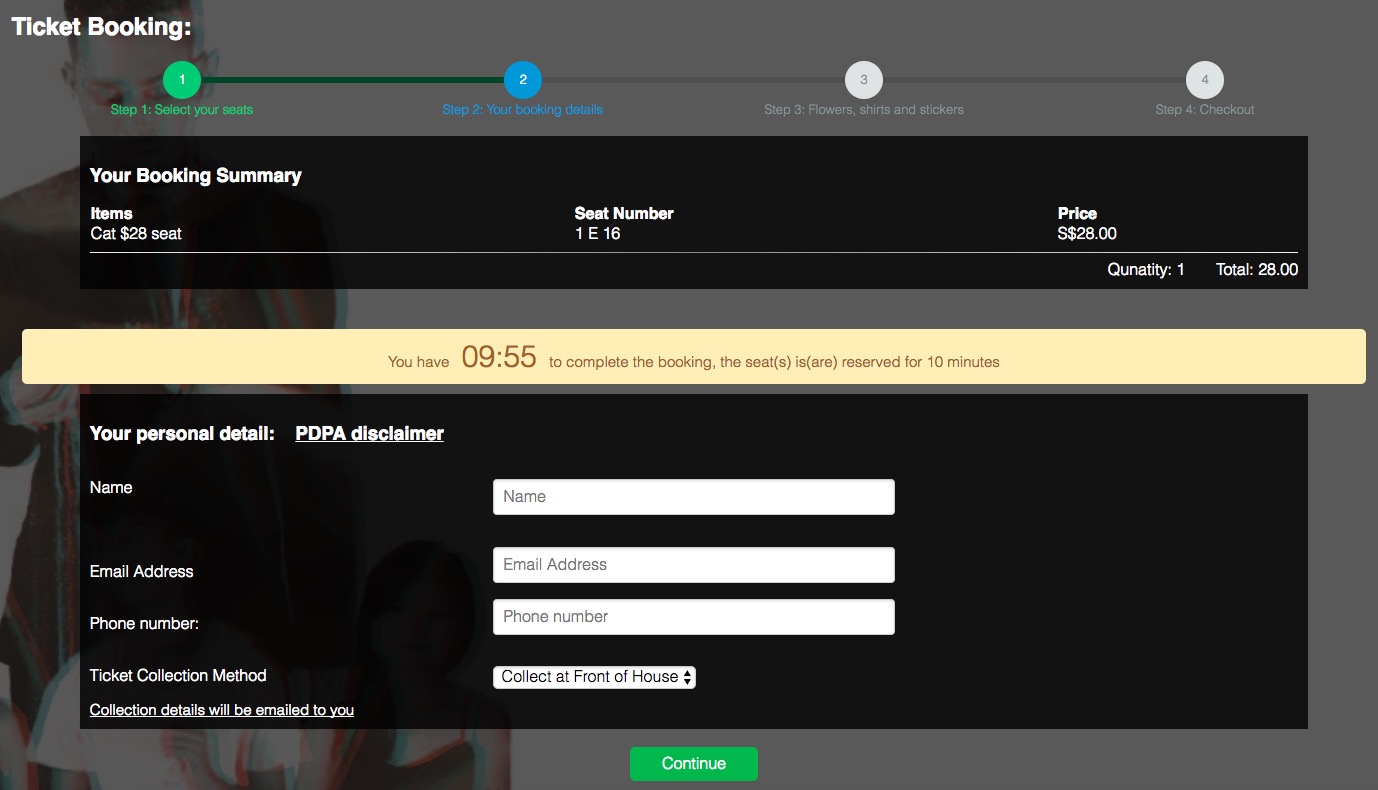
Collect information and start the blocking process.
Step 4
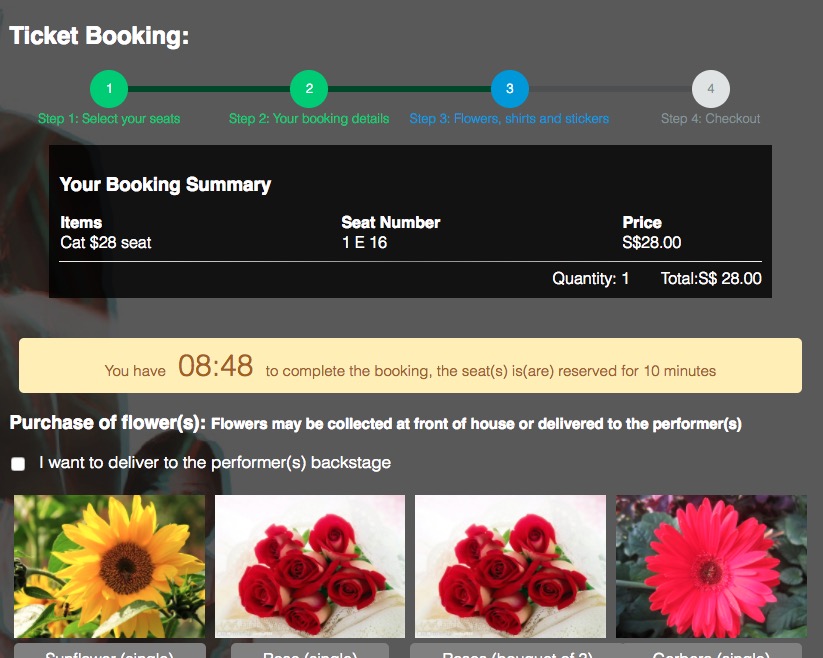
They may want to purchase additional items
Some of items have been marked as “Sold out”.
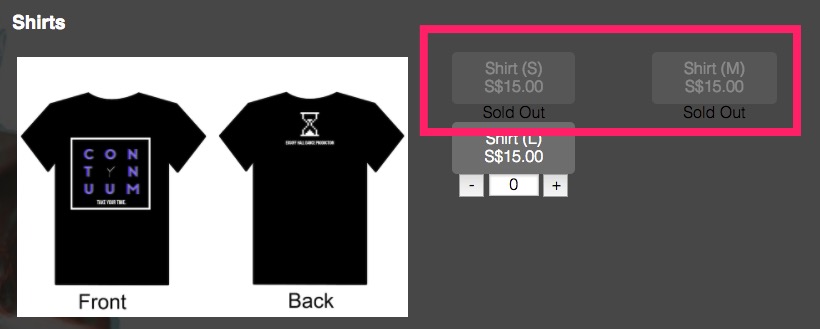
Step 5
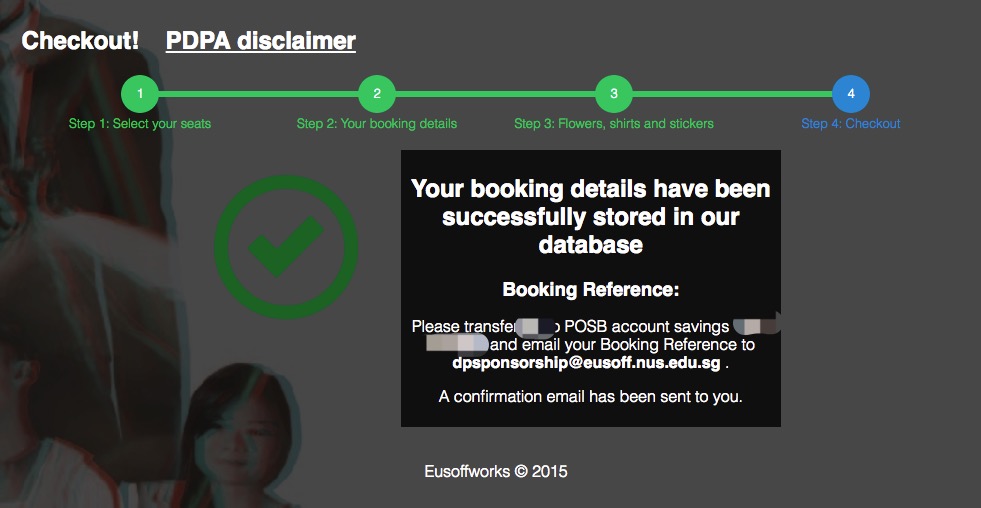
Booking Success and need to transfer money.
Step 6
Admin will need to approve their booking at back-end.
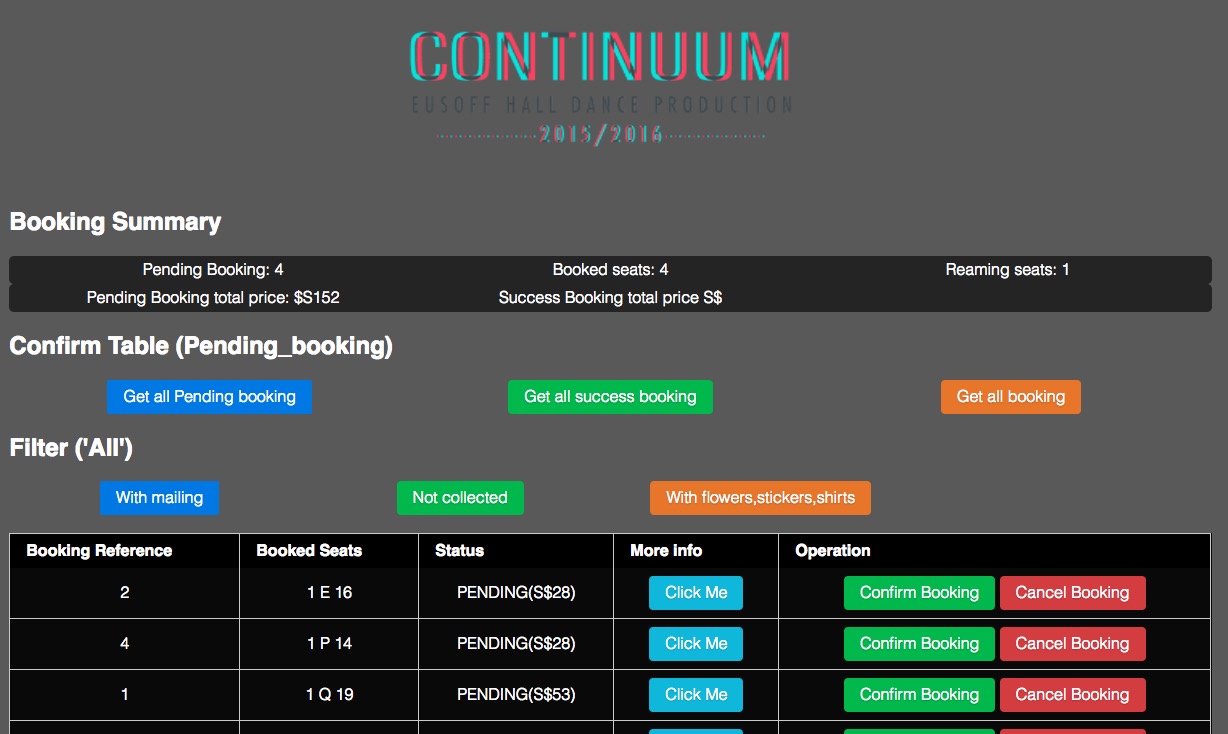
Confirm the booking when money has been transfered.
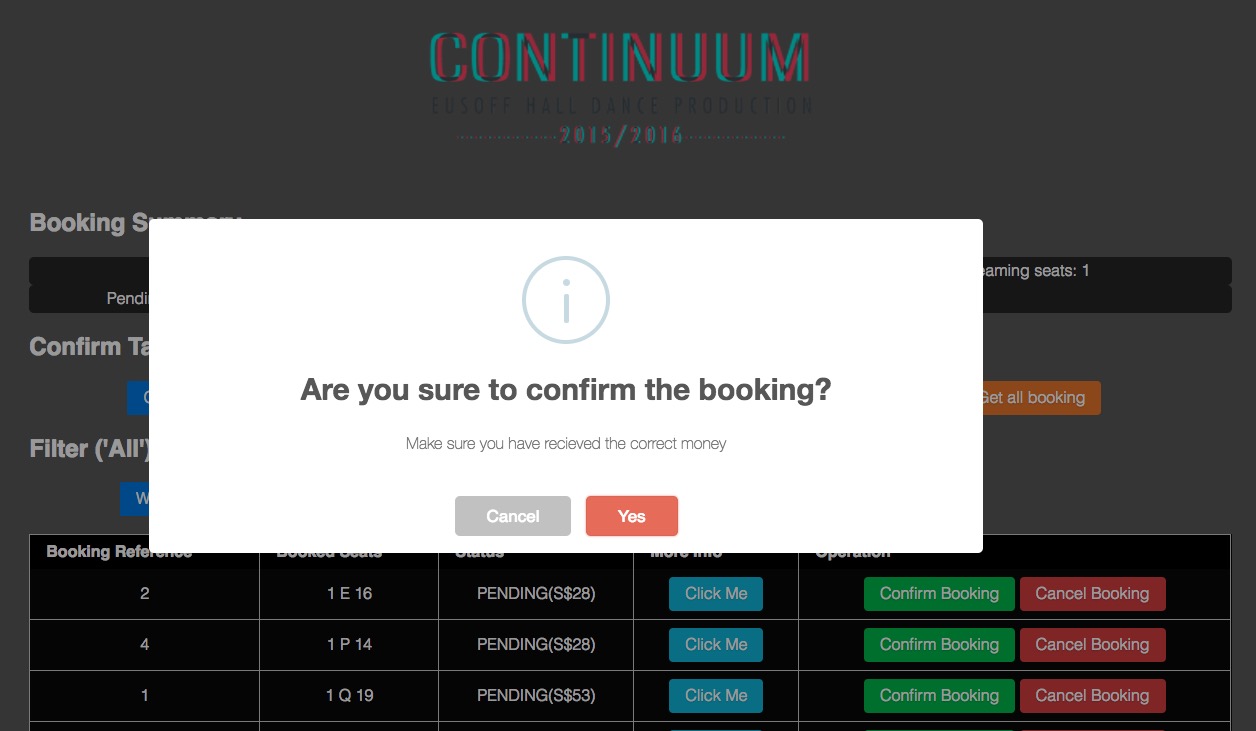
Things that we did right
This is my first project in NUS and it was built during my first semester in the university. At that time, I hadn’t learnt things in software engineering yet. Nevertheless, there are still some good practices that I appreciated.
No magic number
1 | define("DB_HOST", "localhost:8889"); |
We define constant in the file constant.php
and include
it when necessary in every files. This is pretty good as there is no magic number inside the PHP script.
Dedicated file for interaction with the database
1 |
|
In a common file called db.php
, we define all the method we need for interaction with database. Though at that time, I don’t really learn OOP programming. This practice is fair enough for abstraction.
Things that need improvements
Not very good code quality
Let’s take a look at the code for checking “No empty seat between booked seat”
1 | function check_seat_between(sc, all_seat, floor, ignore_id){ |
I appreciate that we have good comments on this. But we could absolutely do better for single level of abstraction and separation of concerns. For example, the code for toggling the UI should not be put here.
Hacking Solutions
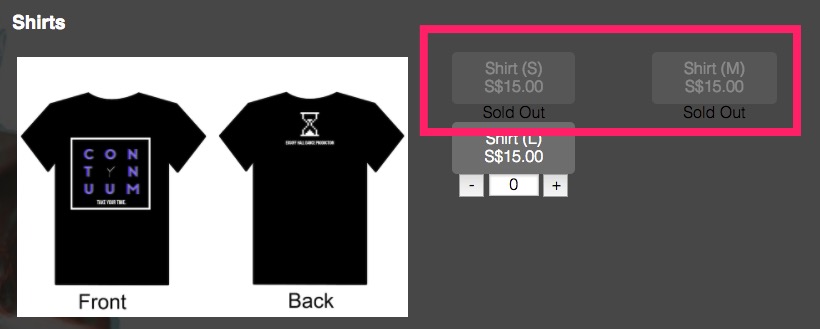
We actually disable the button manually by adding disable
to element. This can definitely don in database.
Manual testing
There is no testing concept at that time when doing this project. Everything is done manually (by visiting the local server to see if it works). And therefore, this results in LIVE issues.
LIVE issues
We forget to check the availability of seats in the back-end and result in double booking (two different person got the same seat). In this case, we have to manually negotiate with the person. This lessen also tells us how testing is important. For such system, testing for concurrent requests is necessary.
Seat Booking System: Eusoff DP1516
http://puxiao.me/School-Project/Booking-System/DP1516/2018-01-27-Seat-Booking-System-Eusoff-DP1516/